In Chapter 14 of Python Tutorial, we will learn about Modules and Packages
Before we begin, if you are viewing this tutorial on Mobile, please rotate your mobile horizontally for best experience. You can enable auto-rotate in the phone settings.
In Python, module is a simple file having .py extension and it contains set of functions, classes, or variables defined and implemented. With module, we can organize our code into reusable units by grouping related code into a single file.
Module can be imported into other Python scripts using the built-in import statement, which allows us to use the functions and classes defined in the module.
Let’s say we want to create a game application: the game application is going to need one module for the game logic, and another module that displays the game on the screen, and so on. Each module consists of a different file, which may be edited separately.
We can put all the game application code in one file, but then the code becomes very large, hard to maintain and unreadable.
By using modules, it is easy to break down the code into smaller chunks of code that are more manageable. We can put the game logic code in one file, code for displaying game on screen in another file, and so on. Breaking the code down into these different modules promotes organization, reusability, and maintainability.
Let’s create a module by creating a file, give it a name and a .py extension:
#demo.py
# creating a variable in the module
demo_var = "variable created in this module"
# A function in the module
def greeting(name):
return("Hello %s, How are you?" %name)
# creating another function in the module
def add(a, b):
result = a + b
return result
print(demo_var)
print(greeting("Alex"))
print(add(2, 5))
The code above defines a module named demo.py. This module contains a variable named demo_var whose value is the string “variable created in this module”. This module also contains two functions. When called, the greeting() function takes in a name parameter, and it prints a greeting message if we pass a name to it. The add() function returns the sum of two numbers that have been passed to it.
Now, for example, you want to use variables, functions, and classes defined in one module in another module, you would need to import them. There are different ways of importing modules, let’s check them out.
Using the import statement:
By using import statement, we make the contents of one module available for use in another module. Check the example below:
#creating another module with the name demo_two.py import demo print(demo.demo_var) print(demo.greeting(“Alex”)) print(demo.add(2, 5)) #output variable created in this module Hello Alex, How are you? 7
In the example above, functions and variable from the demo.py module were imported and made available for use in demo_two.py. We don’t include the .py extension when using import statement; Python automatically knows we’re importing a module.
Using the from keyword:
Instead of importing entire module, if you want to import specific functions or variables then, use the from keyword. This will be really helpful when there are large number of functions are defined in the module. Let’s check the below example:
# demo_from.py from demo import add print(add(5, 7))
As we required only add function from the demo module, we imported it using the from keyword.
You may have noticed that in the above example, the name of the module does not precede add function, because we’ve specified the module name using the import command. This helps in making the code more concise and readable.
Import all from a module:
We can use import all objects in a module by using import * command. Check the below example:
# demo_all.py from demo import * print(demo_var) print(add(4,5))
Using as keyword
Modules can be imported under any name you want. Using as keyword, we can provide alias or an alternate name for the module. Check the below example:
# demo_as.py import demo as dem result = dem.add(7, 9) print(result) print(dem.greeting("Alex"))
Built-in Modules:
Using dir() built-in function, we can list all the functions in the module. Another important function, which might come in handy, is help().
Let’s use see dir() in an example:
import math
dir(math)
Please note that math is a built-in module. Here, we can see a sorted list of names from the math module. All names that begin with an underscore are default Python attributes associated with the module (these are not user-defined).
Now, if there is a function in the module for which you want to know what is it about or what does it do, then by using help function we can get that information. Check the example below:
import math
help(math.tan)
Packages:
In Python, packages are collection of modules, sub-packages that contain various set of functions. These modules and sub-packages are organized in a directory hierarchy.
Working on huge projects requires us to deal with a lot of code, and having everything in one file will make the code appear messy. To overcome this challenge, we can keep related code together in packages and divide our code into several files.
With packages, we can organize our related code modules or sub-packages in one directory however, just putting the related code in a directory doesn’t make it a package. We need a special file called init.py.
This __init__.py file can be an empty file. It indicates that the directory it’s in is a Python package or a sub-package. When this file is available in the directory then, we can import packages or sub-packages the same way as we import a module.
The init.py file is mostly empty, but it contains the initialization code for the corresponding package or sub-package. For this reason, this file is vital in Python’s package structure and import mechanisms.
Earlier, we have taken game application to explain how module works. Let’s take a similar example for package. For example, in a gaming application, you are dealing with three services – Logic, Sound and Level
Let us suppose we have the following modules in the application:
- draw.py
- start.py
- load.py
- stage.py
- mute.py
- close.py
- open.py
- pause.py
- play.py
Now, imagine if we put these modules into single file, it will be lot of code, hard to maintain and creates lot of confusion. Therefore, it is better to divide our code into several files and organize it in a directory hierarchy.
Graphical Representation of Package Model Structure:
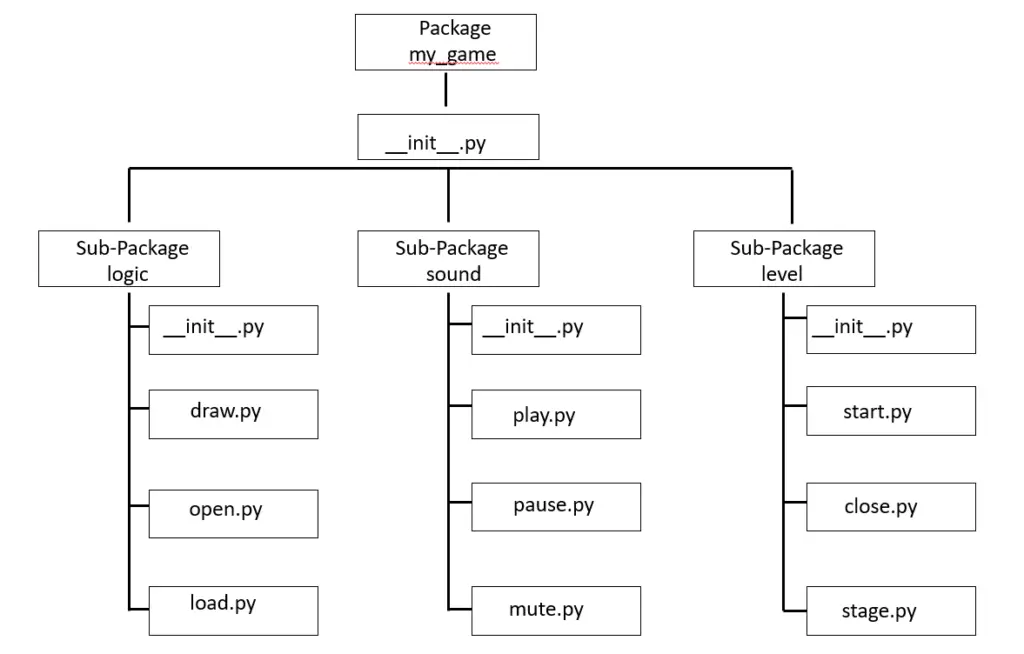
From above package, if we want to import stage module, we can do it in two ways:
import game.level.stage
If there is a function in this module say difficulty then, to access this function we have to use the full prefix:
game.level.stage.difficulty
If you don’t like using the full prefix for access functions in the module then, we can import module without package prefix as below:
from game.level import stage
Now, to call the function simply do as below:
stage.difficulty()
That brings to the end of chapter 14 of Python tutorial, see you in the next tutorial!!!
By the way, if you haven’t installed Python and Pycharm IDE on your Windows laptop, you can visit the below links to learn on how to install and configure/install Python and Pycharm IDE on Windows OS. This might be helpful in understanding the Python language easier.
How to Install Python 3 and Pycharm IDE on Windows
How to configure Pycharm IDE and “Run” your first Python 3 program
For your reference, to download Pycharm IDE, visit below link:
https://www.jetbrains.com/pycharm/download/?section=windows
However, if you don’t want to go through installation process, you can practice your learnings on this page itself by using integrated Python IDE above.
If you have any questions or comments about chapter 14 of Python tutorial, please let us know in the comments section below.